- PHP Basics
- Learn PHP
- PHP Comments
- PHP Data Types
- PHP Variables
- PHP Operators
- PHP echo
- PHP print
- PHP echo vs. print
- PHP if else
- PHP switch
- PHP for Loop
- PHP while Loop
- PHP do...while Loop
- PHP foreach Loop
- PHP break and continue
- PHP Arrays
- PHP print_r()
- PHP unset()
- PHP Strings
- PHP Functions
- PHP File Handling
- PHP File Handling
- PHP Open File
- PHP Create a File
- PHP Write to File
- PHP Read File
- PHP feof()
- PHP fgetc()
- PHP fgets()
- PHP Close File
- PHP Delete File
- PHP Append to File
- PHP Copy File
- PHP file_get_contents()
- PHP file_put_contents()
- PHP file_exists()
- PHP filesize()
- PHP Rename File
- PHP fseek()
- PHP ftell()
- PHP rewind()
- PHP disk_free_space()
- PHP disk_total_space()
- PHP Create Directory
- PHP Remove Directory
- PHP Get Files/Directories
- PHP Get filename
- PHP Get Path
- PHP filemtime()
- PHP file()
- PHP include()
- PHP require()
- PHP include() vs. require()
- PHP and MySQLi
- PHP and MySQLi
- PHP MySQLi Setup
- PHP MySQLi Create DB
- PHP MySQLi Create Table
- PHP MySQLi Connect to DB
- PHP MySQLi Insert Record
- PHP MySQLi Update Record
- PHP MySQLi Fetch Record
- PHP MySQLi Delete Record
- PHP MySQLi SignUp Page
- PHP MySQLi LogIn Page
- PHP MySQLi Store User Data
- PHP MySQLi Close Connection
- PHP Misc Topics
- PHP Object Oriented
- PHP new Keyword
- PHP Cookies
- PHP Sessions
- PHP Date and Time
- PHP GET vs. POST
- PHP File Upload
- PHP Image Processing
Getting Started with PHP
Here you will see how to get started in PHP programming, or how to program in PHP language.
How PHP Program can be Written ?
Let's take a simple program in PHP to see how PHP program can be writted.
<html> <head> <title>Getting Started with PHP Programming - fresherearth</title> </head> <body> <?php echo "<h2>Getting Started with PHP Programming</h2>"; echo "<p>Let's get started with world class scripting language.</p>"; echo "<p>Or let's get started with PHP programming.</p>"; ?> </body> </html>
The above PHP code will produce the output given here:
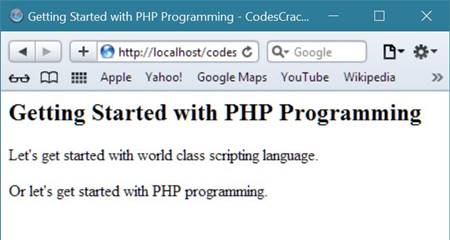
Let's take another PHP simple example printing some paragraphs.
<html> <head> <title>Getting Started with PHP - fresherearth</title> </head> <body> <?php echo "<p>You can either use echo or print to "; echo "to output the text.</p>"; echo "<p>Hello World</p>"; print "<p>Hello World</p>"; ?> </body> </html>
Here is the sample output produced by the above PHP code:
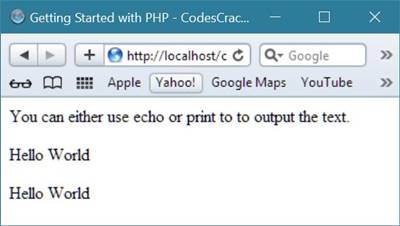
« Previous Tutorial Next Tutorial »