- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Environment Setup
Compile and Run Java Program
To compile and run your Java program, you have to first setup environment for Java programming. You can setup environment for Java without any software, just by using your computer's command prompt. But this is time taking environment. It means if you compile and run your Java program using command prompt, this consumes your extra time for your each and every Java program.
So you can easily use Java compiler/IDE like Netbean or BlueJ to compile and run your Java program very easily. These two Java compiler/IDE are the most popular Java compiler/IDE.
Let's see how to setup environment using Netbean and BlueJ and also using command prompt(cmd).
Java Environment Setup - BlueJ
To compile and run your Java program using BlueJ, follow the following steps :
1. Download Java JDK from Internet and Install it.
2. Now download BlueJ from Internet and Install it.
3. After installing BlueJ, Open it.
4. The Opened BlueJ window will look like :
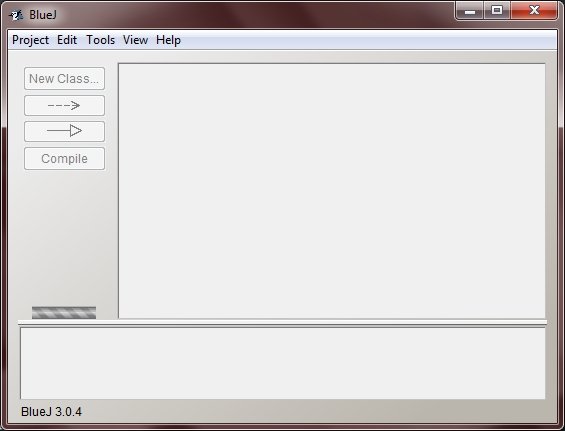
5. Now Click on the Project Menu then New Project present at the left top of the BlueJ window as shown in the following figure :
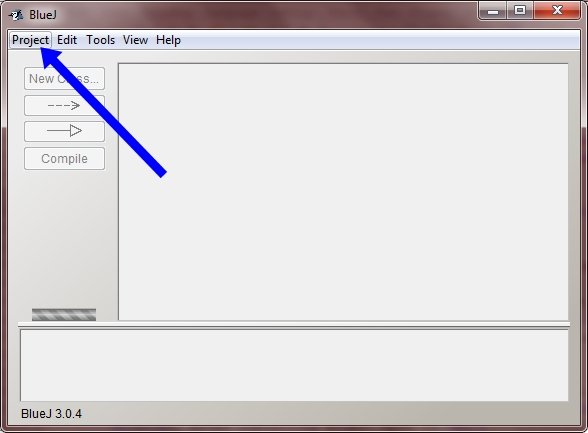
6. Now Type Project Name like JavaProgram.java and click create or simply press enter as shown in the following figure :
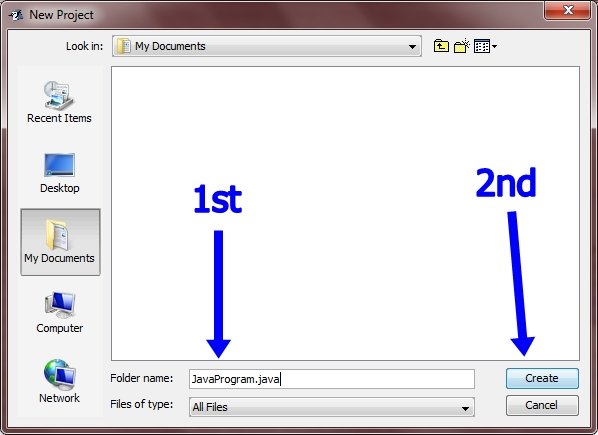
7. After performing this action, you will watch some changes in BlueJ window as shown in the following figure:
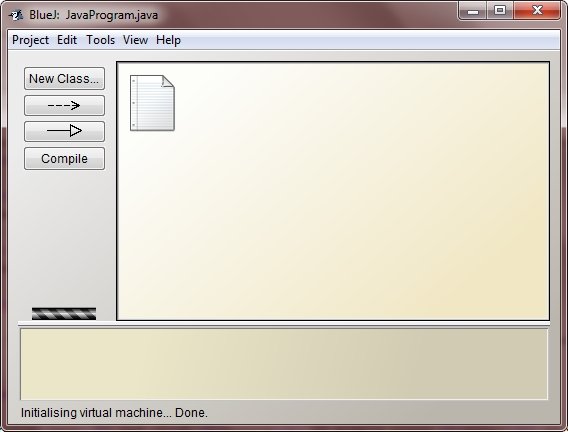
8. Now Click on the New Class Menu and Type Class name like JavaProgram then click OK button as shown in the following figure :
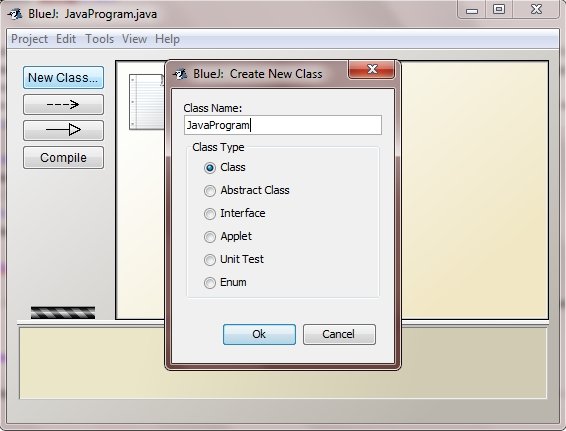
9. After performing this action, you will watch again some changes in BlueJ window as shown in the following figure:
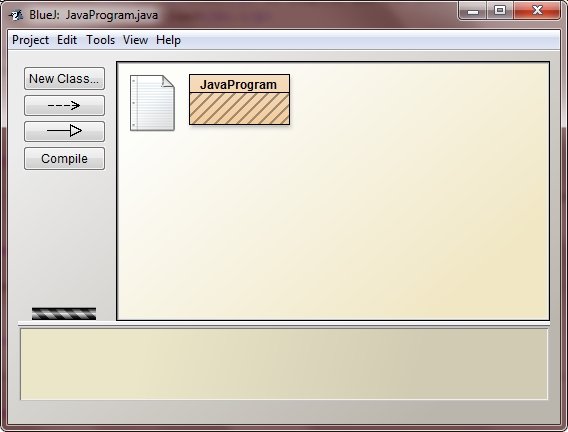
10. Now press double click on JavaProgram or make right click on JavaProgram and then click on Open Editor then a new Editor window will come out that will look like :
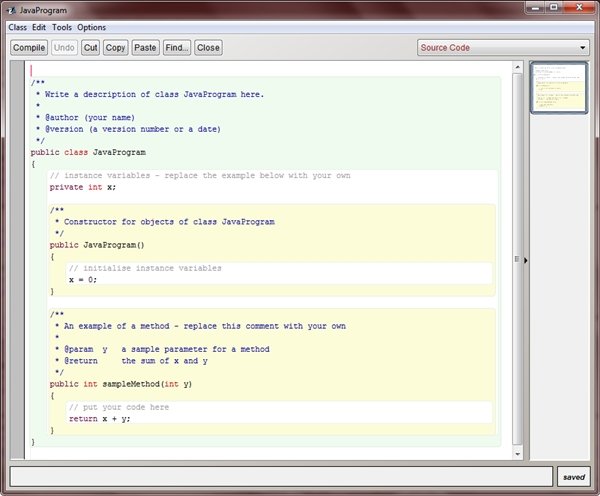
11. Now remove all the codes/texts that already available in the window and start typing your own Java program like :
public class JavaProgram { public static void main(String args[]) { System.out.println("Hello BlueJ, I am Java"); } }
as shown in the following figure :
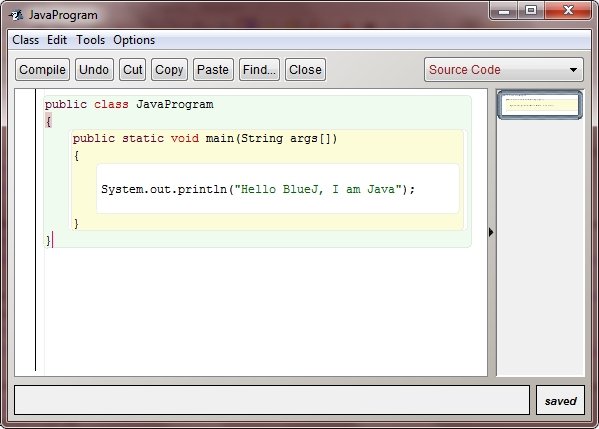
12. Now press Compile button, now you will watch some message at the bottom of the window as shown in the following figure :
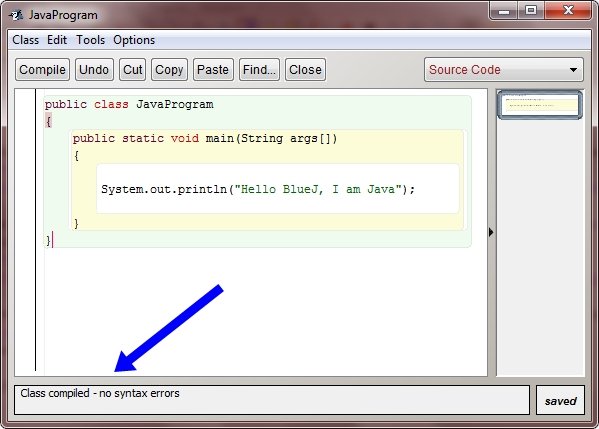
13. If you will watch, no syntax errors, then close the window by pressing the close button
14. Now you will come to the back window, if you want to run and check your Java program's output, then right click on the class i.e. on JavaProgram and then click void main(String[] args), now a small window will come out like :
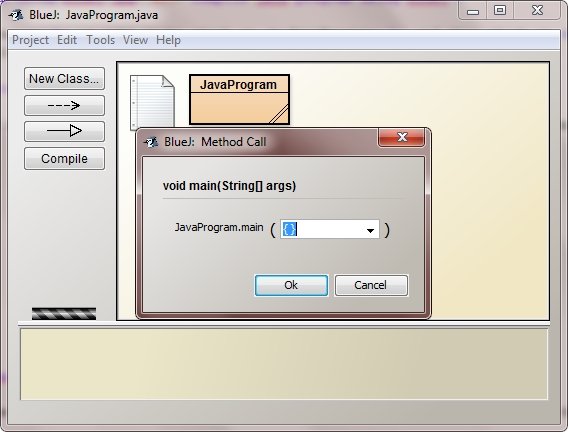
15. Now press OK button to watch the output of your Java program, as shown in the following figure:
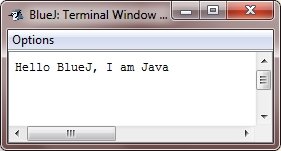
Java Environment Setup - Netbean
To compile and run your Java program using Netbean, follow the following steps :
1. Download Java JDK from Internet and Install it.
2. Now download Netbean from Internet and Install it.
3. After installing Netbean, Open it.
4. The Opened Netbean window will look like :
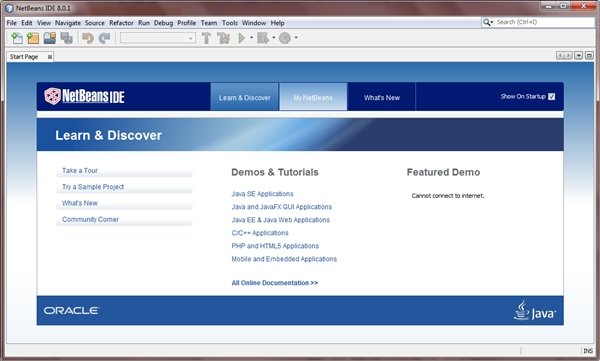
5. Now Click on File Menu then New Project present at the top left of the window, now a new window will come out as shown in the following figure :
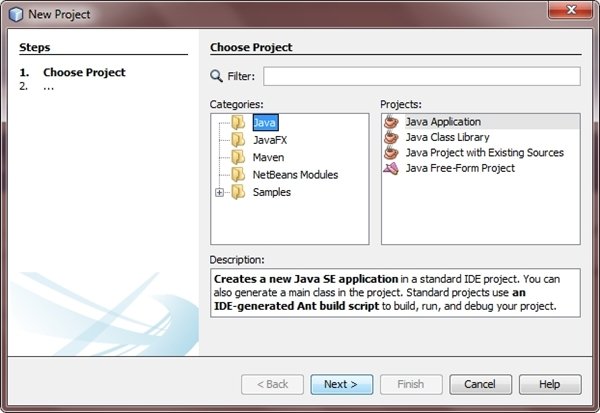
6. Now click on the Next button, then a new window again will come out as shown in the following figure :
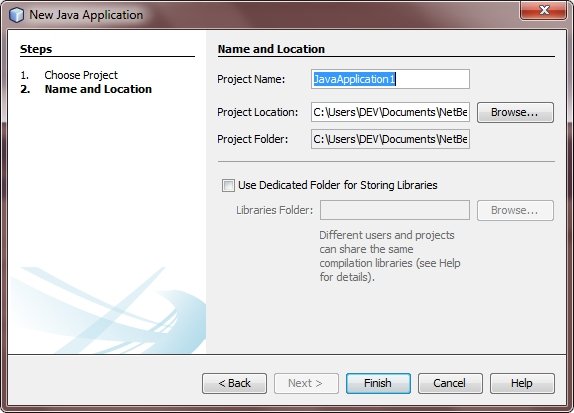
7. Now, you can type your project name or simply leave it default name like JavaApplication1 and press Finish button, after pressing the Finish button, your project is created, and a new window will come out (i.e. editor window) as shown in the following figure:
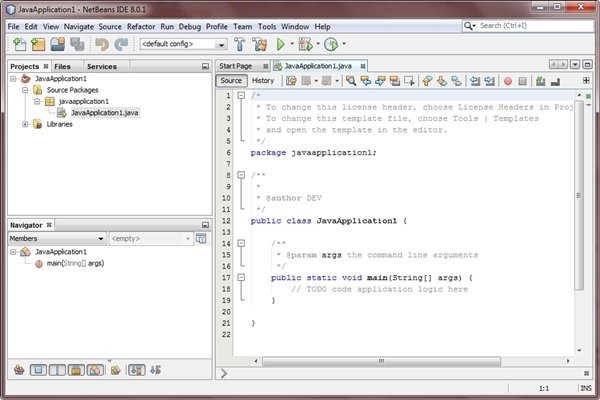
8. Now you can type your Java programming code after the main() function i.e. below the // TODO code application logic here, you can also delete all the pre written codes/texts and write fresh code after removing unusable texts as shown in the following figure :
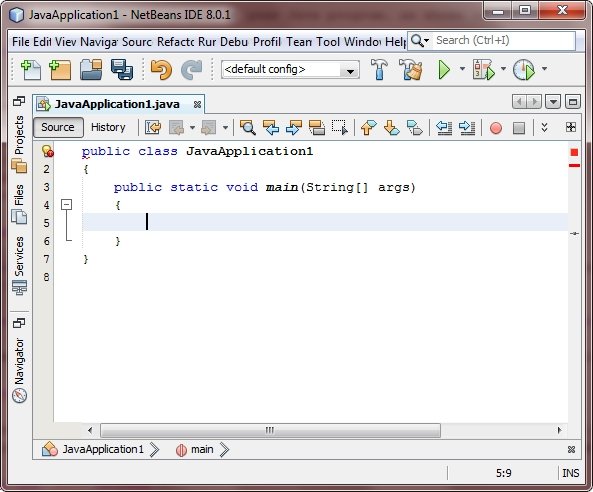
9. Now type your Java program like :
public class JavaApplication1 { public static void main(String[] args) { System.out.println("Hello Netbean!, I am Java"); } }
as shown in the following figure:
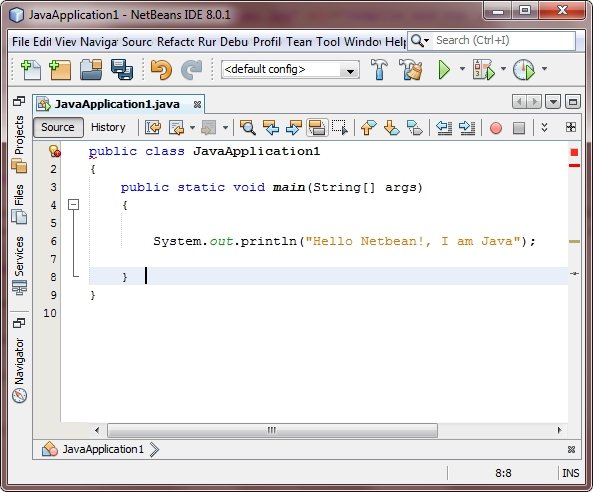
10. After typing your Java program, you can compile by pressing F9 button and run by pressing F6 button or you can also compile your Java program by clicking on the run menu present at the top of the Netbean window. After compiling and running the above Java programming code, you will watch the output as shown in the following figure:
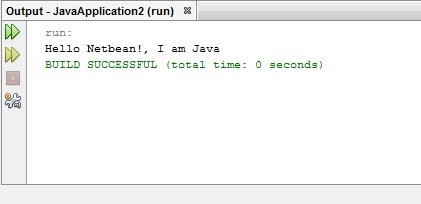
Compile and Run Hello World Java Program using Command Prompt
Java's simplest program which would print the string "Hello World" as output.
/* Java Program Example - Java Environment Setup * This program prints "Hello World" */ public class JavaProgram { public static void main(String args[]) { System.out.println("Hello World"); // prints Hello World } }
Java Environment Setup - Command Prompt
To compile and run the above Java program using the command prompt, let's see how to do this.
Let's explain how to save the file, and compile then run the program using the text editor (Notepad):
- Copy and paste the above code in the Notepad (Windows text editor).
- Save the file with name JavaProgram.java.
- Now open the command prompt window (for windows user) just by pressing the windows key then type cmd and press the ENTER button, and go to the directory where you saved the class. Now let's assume it is in the C:\ directory.
- Type 'javac JavaProgram.java' and press the ENTER button to compile your code. If there will no errors in your code, then the command prompt will take you to the next line (Assume that the path variable is already set).
- Type 'java JavaProgram' to run your Java program.
- After performing this, it will print the 'Hello World' on the window.
Here is the output produced by the above Java program, after typing the first two line:
C : > javac JavaProgram.java C : > java JavaProgram Hello World
« Previous Tutorial Next Tutorial »