- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java switch Statement
The switch statement is Java's multi-way branch statement. The switch statement provides an easy way to dispatch the execution to different parts of your code based on the value of an expression. As such, it often provides a better alternative than a large series of the if-else-if statements.
Here is the general form to use switch statement in Java:
switch(expression) { case value1: // statement sequence break; case value2: // statement sequence break; . . . case valueN: // statement sequence break; default: // default statement sequence }
For versions of Java prior to JDK 7, expression must be of the type byte, short, int, char, or an enumeration (you will learn later about enumerations in separate chapter).
Beginning with JDK 7, expression can also be of the type String. Each value specified in the case statements must be a unique constant expression (such as a literal value). Duplicate case values are not allowed. The type of each value must be compatible with the type of expression.
Java switch Statement Working
The switch statements works like this:
The vale of the expression is compared with each of the values in case statements. If a match is found, then the code sequence following
that case statement is executed. If none of the constants matches the value of the expression, then the default statement
is executed. However, the default statement is optional. If no case matches and no default is present,
then no further action is taken.
The break statement is used inside the switch to terminate a statement sequence. When a break statement is encountered, execution branches to the first line of code that follows the entire switch statement. This has the effect of "jumping out" of the switch statement.
Java switch Statement Example
Following is a simple example that uses a switch statement :
/* Java Program Example - Java switch Statement * A simple example of the switch statement */ public class JavaProgram { public static void main(String args[]) { int i; for(i=0; i<10; i++) { switch(i) { case 0: System.out.println("i is zero."); break; case 1: System.out.println("i is one."); break; case 2: System.out.println("i is two."); break; case 3: System.out.println("i is three."); break; case 4: System.out.println("i is four."); break; case 5: System.out.println("i is five."); break; default: System.out.println("i is greater than 5."); } } } }
When the above Java program is compile and executed, it will produce the following output:
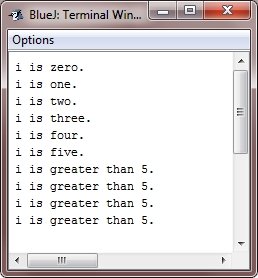
As you can see, each time through the loop, the statements associated with the case constant that matches i are executed. All the others are bypassed. After i is greater than 5, no case statements match, so the default statement is executed.
The break statement is optional. If you omit the break, execution will continue on into the next case. If sometimes desirable to have multiple cases without break statements between them. For example, consider the following program :
/* Java Program Example - Java switch Statement * In a switch, break statement are optional */ public class JavaProgram { public static void main(String args[]) { int i; for(i=0; i<15; i++) { switch(i) { case 0: case 1: case 2: case 3: case 4: System.out.println("i is less than 5."); break; case 5: case 6: case 7: case 8: case 9: System.out.println("i is less than 10."); break; default: System.out.println("i is 10 or more"); } } } }
When the above Java program is compile and executed, it will produce the following output:
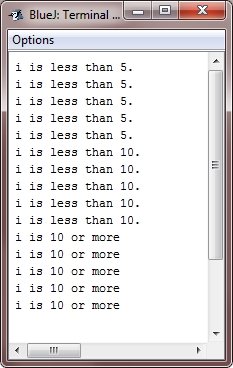
As you can see, execution falls through each case until a break statement (or the end of the switch) is reached.
While the preceding example is, of course, contrived for the sake of illustration, omitting the break statement has many practical applications in real programs. To sample its more realistic usage, consider the following rewritten of the season example shown earlier (in the Java if statement chapter). This version uses a switch to provide a more efficient implementation.
/* Java Program Example - Java switch Statement * An improved version of the season program shown earlier */ public class JavaProgram { public static void main(String args[]) { int month = 4; String season; switch(month) { case 12: case 1: case 2: season = "Winter"; break; case 3: case 4: case 5: season = "Spring"; break; case 6: case 7: case 8: season = "Summer"; break; case 9: case 10: case 11: season = "Autumn"; break; default: season = "Bogus Month"; } System.out.println("April is in the " + season); } }
When the above Java program is compile and executed, it will produce the following output:
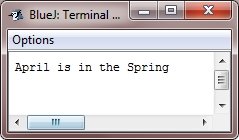
As mentioned, beginning with JDK 7, you can use a string to control a switch statement. For example:
/* Java Program Example - Java switch Statement * Use a string to control a switch statement */ public class JavaProgram { public static void main(String args[]) { String str = "two"; switch(str) { case "one" : System.out.println("one"); break; case "two" : System.out.println("two"); break; case "three" : System.out.println("three"); break; default : System.out.println("no match found.!!"); break; } } }
When the above Java program is compile and executed, it will produce the following output:
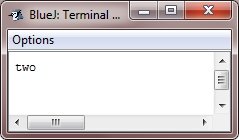
The string contained in str (which is "two" in this program) is tested against case constants. When a match is found (as it is in the second case), the code sequence associated with that sequence is executed.
Being able to use the strings in a switch statement streamlines many situations. For example, using a string-based switch is an improvement over the equivalent sequence of if/else statements. However, switching on the strings can be more expensive than switching on the integers. Therefore, it is best to switch on strings only in cases in which the controlling data is already in the string form. In other words, don't use string in a switch unnecessarily.
Java Nested switch
You can use a switch as part of the statement sequence of an outer switch. This is called a nested switch.
Since a switch statement defines its own block, no conflicts arise between the case constants in the inner switch and those in the outer switch. For example, the following code fragment is perfectly valid :
case(count) { case 1: switch(target) // nested switch { case 0: System.out.println("target is zero."); break; case 1: // no conflicts with outer switch System.out.println("target is one."); break; } break; case 2: // ...
Here, the case 1: statement in the inner switch does not conflict with the case 1: statement in the outer switch. The count variable is compared only with the list of cases at the outer level. If count is 1, then the target is compared with the inner list cases.
In summary, there are the following three important features of the switch statement to note :
- The switch differs from the if in that switch can only test for equality, whereas if can evaluate any type of Boolean expression. That is, the switch looks only for a match between the value of the expression and one of its case constants.
- No two case constants in the same switch can have identical values. Of course, a switch statement and an enclosing outer switch can have case constants in common.
- A switch statement is usually more efficient than a set of nested ifs.
The last point is particularly interesting because it gives insight into how the Java compiler works. When it compiles a switch statement, the Java compiler will inspect each of the case constants and create a "jump table" that it will use for selecting the path of execution depending on the value of the expression.
Therefore, if you need to select among a large group of values, a switch statement will run much faster than the equivalent logic code using a sequence of if-elses. The compiler can do this because it knows that the case constants are all the same type and simply must be compared for equality with the switch expression. The compiler has no such knowledge of a long list of the if expressions.
« Previous Tutorial Next Tutorial »