- Java Programming Basics
- Java Tutorial
- Java Overview
- Java Environment Setup
- Java Program Structure
- Java Basic Syntax
- Java First Program
- Java Constants
- Java Separators
- Java Keywords
- Java Data Types
- Java Data Types
- Java Integers
- Java Floating Point
- Java Characters
- Java Booleans
- Java Numbers
- Java Programming Variables
- Java Variables
- Java Variable Types
- Java Variable Scope
- Java Type Conversion
- Java Type Casting
- Java Auto Type Promotion
- Java Type Promotion Rules
- Java Programming Arrays
- Java Arrays
- Java One Dimensional Array
- Java Multidimensional Array
- Java Programming Operators
- Java Operators
- Java Arithmetic Operators
- Java Increment Decrement
- Java Bitwise Operators
- Java Left Shift
- Java Right Shift
- Java Relational Operators
- Java Boolean Logical Operators
- Java Ternary(?) Operator
- Java Operator Precedence
- Java Control Statements
- Java Decision Making
- Java if if-else if-else-if
- Java switch Statement
- Java Loops
- Java while Loop
- Java do-while Loop
- Java for Loop
- Java for-each Loop
- Java Nested Loops
- Java break Statement
- Java continue Statement
- Java Class Object Method
- Java Classes and Objects
- Java Class
- Java Object
- Java new Operator
- Java Methods
- Java Constructors
- Java this Keyword
- Java Stack
- Java Overloading Recursion
- Java Method Overloading
- Java Constructor Overloading
- Java Object as Parameter
- Java Call by Value Reference
- Java Returning Objects
- Java Recursion
- Java Modifier Types
- Java Encapsulate Poly String
- Java Encapsulation
- Java Polymorphism
- Java Nested Inner Class
- Java Strings
- Java Command Line Arguments
- Java Variable Length Arguments
- Java Inheritance Abstraction
- Java Inheritance
- Java super Superclass
- Java Multilevel Hierarchy
- Java Method Overriding
- Java Abstraction
- Java Packages Interfaces
- Java Packages
- Java Access Protection
- Java Import Statement
- Java Interfaces
- Java Programming Exceptions
- Java Exception Handling
- Java try catch
- Java throw throws
- Java finally Block
- Java Built In Exceptions
- Java Exception Subclasses
- Java Chained Exceptions
- Java Multithreading
- Java Multithreading
- Java Thread Model
- Java Main Thread
- Java Create Thread
- Java Thread Priorities
- Java Synchronization
- Java Inter Thread Communication
- Java Suspend Resume Stop Thread
- Java Get Thread State
- Java Enum Autobox Annotation
- Java Enumerations
- Java Type Wrappers
- Java Autoboxing
- Java Annotation
- Java Marker Annotations
- Java Single Member Annotation
- Java Built In Annotations
- Java Type Annotations
- Java Repeating Annotations
- Java Data File Handling
- Java Files I/O
- Java Streams
- Java Read Console Input
- Java Write Console Output
- Java PrintWriter Class
- Java Read Write Files
- Java Automatically Close File
- Java Programming Advance
- Java Date and Time
- Java Regular Expressions
- Java Collections Framework
- Java Generics
- Java Data Structures
- Java Network Programming
- Java Serialization
- Java Send Email
- Java Applet Basics
- Java Documentation
- Java Programming Examples
- Java Programming Examples
Java Left Shift Operator
The left shift operator, <<, simply shifts all of the bits in a value to the left, a specified number of times. Here is the general form to use left shift operator in Java:
value << num
Here, num specifies the number of positions to left-shift the value in value i.e., the << simply moves all of the bits in the specified value to the left by the number of bit positions specified by num. For each shift left, the high-order bit is simply shifted out (and lost), and a zero is brought in on the right. This means, when a left shift is applied to an int operand, bits are lost once they are shifted past bit position 31. If the operand is a long, bits are lost after bit position 63.
Java's automatic type promotions produce unexpected results when you are shifting the byte and short values.
As you know, byte and short values are promoted to int when an expression is evaluated.
Moreover, the result of such expression is also an int. This means that the outcome of a left shift on byte or short value will simply be an int, and the bits shifted left will not be lost until they shift past bit position 31.
Moreover, a negative byte or short value will be sign-extended when it is promoted to int. Therefore, the high-order bits will be filled with 1's. For these reasons, to perform a left shift on a byte or short implies that you must eliminate the high-order bytes of the int result.
For example, if you left-shift a byte value, that value will first be promoted to an int and then shifted. It means that you must discard top three bytes of the result when what you want is the result of a shifted byte value. The simplest way to do this is to cast the result back into a byte.
Java Left Shift Operator Example
Here this program illustrates the concept of the left shift operator :
/* Java Program Example - Java Left Shift * Left shifting a byte value. */ public class JavaProgram { public static void main(String args[]) { byte a = 64, b; int i; i = a << 2; b = (byte) (a << 2); System.out.println("Original value of a : " +a); System.out.println("i is " + i + " and b is " + b); } }
When the above Java Program is compile and executed, it will produce the following output:
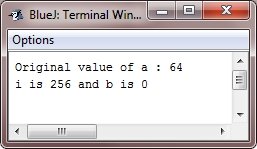
Since a is promoted to int for the purposes of evaluation, and left-shifting the value 64 (0100 0000) twice results in i containing the value 256 (1 0000 0000). However, the value in b contains 0 as after the shift, the low-order byte is now zero. Its only 1 bit has been shifted out.
Since each left shift gives the effect of doubling the original value, programmers frequently uses this fact as an efficient option to multiplying by 2. But you need to watch out. That is, If you shift a 1 bit into the high-order position (bit 31 or 63), the value will become negative. Here this program illustrates this:
/* Java Program Example - Java Left Shift * Left shifting is a quick way to multiply by 2. */ public class JavaProgram { public static void main(String args[]) { int i; int num = 0xFFFFFFE; for(i=0; i<4; i++) { num = num << 1; System.out.println(num); } } }
When the above Java program is compile and executed, it will produce the following output:
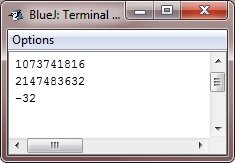
The starting value was carefully chosen because after being shifted left, 4 bit positions, it would produce the value -32. As you can see, when a 1 bit is shifted in bit 31, the number is interpreted as negative.
« Previous Tutorial Next Tutorial »