- JavaScript Basics
- JS Home
- JS Syntax
- JS Placements
- JS Output
- JS Statements
- JS Keywords
- JS Comments
- JS Variables
- JS var
- JS let
- JS const
- JS var Vs let Vs const
- JS Operators
- JS Arithmetic Operators
- JS Assignment Operators
- JS Comparison Operators
- JS Logical Operators
- JS Bitwise Operators
- JS Ternary Operator
- JS Operator Precedence
- JS Data Types
- JS typeof
- JS Conditional Statements
- JS Conditional Statement
- JS if Statement
- JS if...else Statement
- JS switch Statement
- JS Loops
- JS for Loop
- JS while Loop
- JS do...while Loop
- JS break Statement
- JS continue Statement
- JS break Vs. continue
- JavaScript Popup Boxes
- JS Dialog Box
- JS alert Box
- JS confirm Box
- JS prompt Box
- JavaScript Functions
- JS Functions
- JS setTimeout() Method
- JS setInterval() Method
- JavaScript Events
- JS Events
- JS onclick Event
- JS onload Event
- JS Mouse Events
- JS onreset Event
- JS onsubmit Event
- JavaScript Arrays
- JS Array
- JS Find Length of Array
- JS Add Elements at Beginning
- JS Add Element at End
- JS Remove First Element
- JS Remove Last Element
- JS Get First Index
- JS Get Last Index
- JS Reverse an Array
- JS Sort an Array
- JS Concatenate Arrays
- JS join()
- JS toString()
- JS from()
- JS Check if Value Exists
- JS Check if Array
- JS Slice an Array
- JS splice()
- JS find()
- JS findIndex()
- JS entries()
- JS every()
- JS fill()
- JS filter()
- JS forEach()
- JS map()
- JavaScript Strings
- JS String
- JS Length of String
- JS Convert to Lowercase
- JS Convert to Uppercase
- JS String Concatenation
- JS search()
- JS indexOf()
- JS search() Vs. indexOf()
- JS match()
- JS match() Vs. search()
- JS replace()
- JS toString()
- JS String()
- JS includes()
- JS substr()
- JS Slice String
- JS charAt()
- JS repeat()
- JS split()
- JS charCodeAt()
- JS fromCharCode()
- JS startsWith()
- JS endsWith()
- JS trim()
- JS lastIndexOf()
- JavaScript Objects
- JS Objects
- JS Boolean Object
- JavaScript Math/Number
- JS Math Object
- JS Math.abs()
- JS Math.max()
- JS Math.min()
- JS Math.pow()
- JS Math.sqrt()
- JS Math.cbrt()
- JS Math.round()
- JS Math.ceil()
- JS Math.floor()
- JS Math.trunc
- JS toFixed()
- JS toPrecision()
- JS Math.random()
- JS Math.sign()
- JS Number.isInteger()
- JS NaN
- JS Number()
- JS parseInt()
- JS parseFloat()
- JavaScript Date and Time
- JS Date and Time
- JS Date()
- JS getFullYear()
- JS getMonth()
- JS getDate()
- JS getDay()
- JS getHours()
- JS getMinutes()
- JS getSeconds()
- JS getMilliseconds()
- JS getTime()
- JS getUTCFullYear()
- JS getUTCMonth()
- JS getUTCDate()
- JS getUTCDay()
- JS getUTCHours()
- JS getUTCMinutes()
- JS getUTCSeconds()
- JS getUTCMilliseconds()
- JS toDateString()
- JS toLocaleDateString()
- JS toLocaleTimeString()
- JS toLocaleString()
- JS toUTCString()
- JS getTimezoneOffset()
- JS toISOString()
- JavaScript Browser Objects
- JS Browser Objects
- JS Window Object
- JS Navigator Object
- JS History Object
- JS Screen Object
- JS Location Object
- JavaScript Document Object
- JS Document Object Collection
- JS Document Object Properties
- JS Document Object Methods
- JS Document Object with Forms
- JavaScript DOM
- JS DOM
- JS DOM Nodes
- JS DOM Levels
- JS DOM Interfaces
- JavaScript Cookies
- JS Cookies
- JS Create/Delete Cookies
- JavaScript Regular Expression
- JS Regular Expression
- JS RegEx .
- JS RegEx \w and \W
- JS RegEx \d and \D
- JS RegEx \s and \S
- JS RegEx \b and \B
- JS RegEx \0
- JS RegEx \n
- JS RegEx \xxx
- JS RegEx \xdd
- JS RegEx Quantifiers
- JS RegEx test()
- JS RegEx lastIndex
- JS RegEx source
- JavaScript Advance
- JS Page Redirection
- JS Form Validation
- JS Validations
- JS Error Handling
- JS Exception Handling
- JS try-catch throw finally
- JS onerror Event
- JS Multimedia
- JS Animation
- JS Image Map
- JS Debugging
- JS Browser Detection
- JS Security
- JavaScript Misc
- JS innerHTML
- JS getElementById()
- JS getElementsByClassName()
- JS getElementsByName()
- JS getElementsByTagName()
- JS querySelector()
- JS querySelectorAll()
- JS document.write()
- JS console.log()
- JS instanceof
- JavaScript Programs
- JavaScript Programs
JavaScript Location Object
The location object in JavaScript helps in storing the information of current URL of the window object. It is a child object of the window object.
The location object has the following two features:
- Properties
- Methods
JavaScript Location Object Properties
The table given below describes the properties of the Location object in JavaScript.
Property | Description | Example |
---|---|---|
href | represents a string specifying the entire URL | http://jobails.com:80?test.asp?id=1#start |
protocol | represents a string at the beginning of a URL up to the first colon (:), which specifies the method of access to the URL | http: or https: |
host | represents a string consisting of the hostname and port strings | jobails.com:80 |
hostname | represents the server name, subdomain, and domain name of a URL | jobails.com |
port | represents a string specifying the communications port that the server uses | 80 |
pathname | represents a string portion of a URL specifying how a particular resource can be accessed | test.asp |
search | represents a string beginning with a question mark that specifies any query information in an HTTP URL | id=1 |
hash | represents a string beginning with a # specifies an anchor name in an HTTP URL | start |
JavaScript Location Object Methods
The following table lists the methods of the Location object in JavaScript.
Method | Description |
---|---|
assign() | Loads a new document in the browser |
reload() | reloads the current document that is contained in the location.href property |
replace() | replaces the current document with the specified new one, you can not navigate back to the previous document using the browser's Back button |
JavaScript Location Object Example
Here is an example demonstrates location object in JavaScript:
<!DOCTYPE HTML> <html> <head> <title>JavaScript Location Object</title> <script type="text/javascript"> function gotoUrl() { window.location.href = window.document.loctn.ProtocolFld. options[window.document.loctn.ProtocolFld.selectedIndex]. text + document.loctn.HostnameFld.value + document.loctn. PathnameFld.value } </script> </head> <body> <h3>Enter URL in following sections</h3> <form name="loctn" method="post"> <pre>Protocol: <select name="ProtocolFld" size="1"> <option>http://</option> <option>file://</option> <option>javascript:</option> <option>ftp://</option> <option>mailto:</option> </select> </pre> <pre> Hostname: <input type="text" size="20" maxlength="256" name="HostnameFld" value="jobails.com"> </pre> <pre> Pathname: <input type="text" size="20" maxlength="100" name="PathnameFld" value="/"> </pre> <pre> <input type="button" name="Go" value="Go" onclick="gotoUrl()"> </pre> </form> </body> </html>
Here is the sample output of the above JavaScript location object example code:
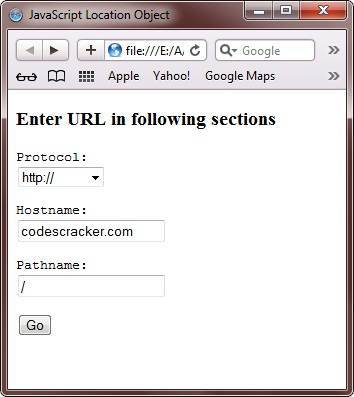
Now fill (or edit and fill) the required field as shown in the following snapshot:
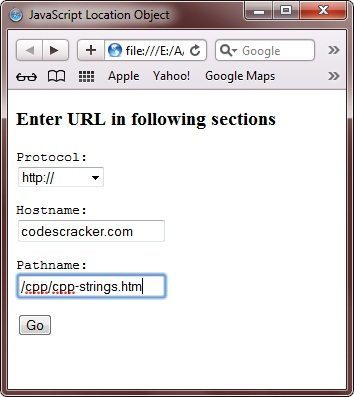
After filling the required field as shown in the above figure, click on Go button to go to that url. Here is the sample output after clicking on the Go button:
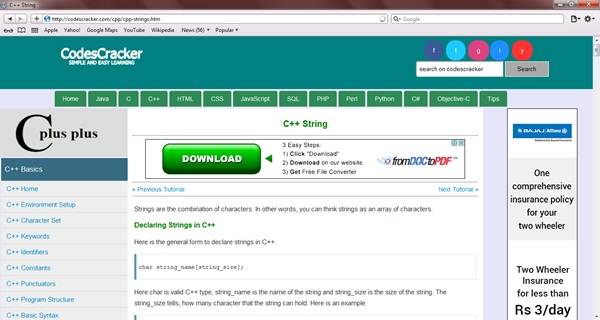
« Previous Tutorial Next Tutorial »