- JavaScript Basics
- JS Home
- JS Syntax
- JS Placements
- JS Output
- JS Statements
- JS Keywords
- JS Comments
- JS Variables
- JS var
- JS let
- JS const
- JS var Vs let Vs const
- JS Operators
- JS Arithmetic Operators
- JS Assignment Operators
- JS Comparison Operators
- JS Logical Operators
- JS Bitwise Operators
- JS Ternary Operator
- JS Operator Precedence
- JS Data Types
- JS typeof
- JS Conditional Statements
- JS Conditional Statement
- JS if Statement
- JS if...else Statement
- JS switch Statement
- JS Loops
- JS for Loop
- JS while Loop
- JS do...while Loop
- JS break Statement
- JS continue Statement
- JS break Vs. continue
- JavaScript Popup Boxes
- JS Dialog Box
- JS alert Box
- JS confirm Box
- JS prompt Box
- JavaScript Functions
- JS Functions
- JS setTimeout() Method
- JS setInterval() Method
- JavaScript Events
- JS Events
- JS onclick Event
- JS onload Event
- JS Mouse Events
- JS onreset Event
- JS onsubmit Event
- JavaScript Arrays
- JS Array
- JS Find Length of Array
- JS Add Elements at Beginning
- JS Add Element at End
- JS Remove First Element
- JS Remove Last Element
- JS Get First Index
- JS Get Last Index
- JS Reverse an Array
- JS Sort an Array
- JS Concatenate Arrays
- JS join()
- JS toString()
- JS from()
- JS Check if Value Exists
- JS Check if Array
- JS Slice an Array
- JS splice()
- JS find()
- JS findIndex()
- JS entries()
- JS every()
- JS fill()
- JS filter()
- JS forEach()
- JS map()
- JavaScript Strings
- JS String
- JS Length of String
- JS Convert to Lowercase
- JS Convert to Uppercase
- JS String Concatenation
- JS search()
- JS indexOf()
- JS search() Vs. indexOf()
- JS match()
- JS match() Vs. search()
- JS replace()
- JS toString()
- JS String()
- JS includes()
- JS substr()
- JS Slice String
- JS charAt()
- JS repeat()
- JS split()
- JS charCodeAt()
- JS fromCharCode()
- JS startsWith()
- JS endsWith()
- JS trim()
- JS lastIndexOf()
- JavaScript Objects
- JS Objects
- JS Boolean Object
- JavaScript Math/Number
- JS Math Object
- JS Math.abs()
- JS Math.max()
- JS Math.min()
- JS Math.pow()
- JS Math.sqrt()
- JS Math.cbrt()
- JS Math.round()
- JS Math.ceil()
- JS Math.floor()
- JS Math.trunc
- JS toFixed()
- JS toPrecision()
- JS Math.random()
- JS Math.sign()
- JS Number.isInteger()
- JS NaN
- JS Number()
- JS parseInt()
- JS parseFloat()
- JavaScript Date and Time
- JS Date and Time
- JS Date()
- JS getFullYear()
- JS getMonth()
- JS getDate()
- JS getDay()
- JS getHours()
- JS getMinutes()
- JS getSeconds()
- JS getMilliseconds()
- JS getTime()
- JS getUTCFullYear()
- JS getUTCMonth()
- JS getUTCDate()
- JS getUTCDay()
- JS getUTCHours()
- JS getUTCMinutes()
- JS getUTCSeconds()
- JS getUTCMilliseconds()
- JS toDateString()
- JS toLocaleDateString()
- JS toLocaleTimeString()
- JS toLocaleString()
- JS toUTCString()
- JS getTimezoneOffset()
- JS toISOString()
- JavaScript Browser Objects
- JS Browser Objects
- JS Window Object
- JS Navigator Object
- JS History Object
- JS Screen Object
- JS Location Object
- JavaScript Document Object
- JS Document Object Collection
- JS Document Object Properties
- JS Document Object Methods
- JS Document Object with Forms
- JavaScript DOM
- JS DOM
- JS DOM Nodes
- JS DOM Levels
- JS DOM Interfaces
- JavaScript Cookies
- JS Cookies
- JS Create/Delete Cookies
- JavaScript Regular Expression
- JS Regular Expression
- JS RegEx .
- JS RegEx \w and \W
- JS RegEx \d and \D
- JS RegEx \s and \S
- JS RegEx \b and \B
- JS RegEx \0
- JS RegEx \n
- JS RegEx \xxx
- JS RegEx \xdd
- JS RegEx Quantifiers
- JS RegEx test()
- JS RegEx lastIndex
- JS RegEx source
- JavaScript Advance
- JS Page Redirection
- JS Form Validation
- JS Validations
- JS Error Handling
- JS Exception Handling
- JS try-catch throw finally
- JS onerror Event
- JS Multimedia
- JS Animation
- JS Image Map
- JS Debugging
- JS Browser Detection
- JS Security
- JavaScript Misc
- JS innerHTML
- JS getElementById()
- JS getElementsByClassName()
- JS getElementsByName()
- JS getElementsByTagName()
- JS querySelector()
- JS querySelectorAll()
- JS document.write()
- JS console.log()
- JS instanceof
- JavaScript Programs
- JavaScript Programs
JavaScript Objects
As you know that the JavaScript language is totally based on objects.
In JavaScript, you have the following two options to create an object:
- by creating a direct instance of object
- by creating an object using a function
A direct instance of an object in JavaScript, is created by using the new keyword. Here is the general form shows how to create an object in JavaScript by creating a direct instance of object:
Obj = new object();
You are free to add properties and methods to an object in JavaScript, just by using the dot (.) followed by a property or method name as shown in the below code fragment:
Obj.name="Deepak"; Obj.branch="CSE"; Obj.rollno=15; Obj.getValue();
From the above code fragment, Obj is the newly created object, name, branch, and rollno are its properties, and getValue() is its method.
You will learn all about Object in JavaScript divided into the following tutorials:
- JavaScript Number Object
- JavaScript Array Object
- JavaScript String Object
- JavaScript Boolean Object
- JavaScript Math Object
- JavaScript RegExp Object
- JavaScript Date Object
JavaScript Object Example
The values are written in name:value pairs (name and value separated by a colon). Here is an example of object in JavaScript
<!DOCTYPE html> <html> <head> <title>JavaScript Object Example</title> </head> <body> <p id="object_para1"></p> <p id="object_para2"></p> <script> var object_var = {firstName:"Aman", lastName:"Tripathi", age:19, eyeColor:"black"}; document.getElementById("object_para1").innerHTML = object_var.firstName + " is " + object_var.age + " years old."; document.getElementById("object_para2").innerHTML = "His eye's color is " + object_var.eyeColor + "."; </script> </body> </html>
Here is the output produced by the above JavaScript Object example program.
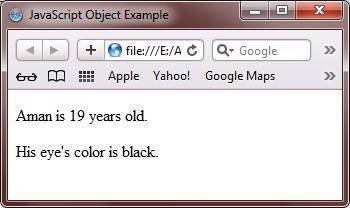
Here is the live demo output produced by the above Object example in JavaScript.
Access Object Properties in JavaScript
Here is an example shows how to access properties of object in JavaScript.
<!DOCTYPE html> <html> <head> <title>JavaScript Object Example</title> </head> <body> <p id="object_para2"></p> <script> var object_var2 = { firstName : "Aman", lastName : "Tripathi", }; document.getElementById("object_para2").innerHTML = object_var2.firstName + " " + object_var2.lastName; </script> </body> </html>
Here is the output produced by the above JavaScript object example.
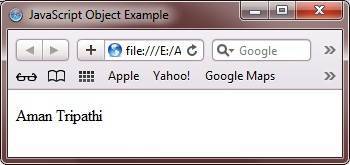
Access Object Methods in JavaScript
Here is an example shows how to access methods of object in JavaScript.
<!DOCTYPE html> <html> <head> <title>JavaScript Object Example</title> </head> <body> <p id="object_para3"></p> <script> var object_var3 = { firstName: "Aman", lastName : "Tripathi", fullName : function(c) { return this.firstName + " " + this.lastName; } }; document.getElementById("object_para3").innerHTML = object_var3.fullName(); </script> </body> </html>
You will watch the same output produced, as in the above example.
« Previous Tutorial Next Tutorial »