- JavaScript Basics
- JS Home
- JS Syntax
- JS Placements
- JS Output
- JS Statements
- JS Keywords
- JS Comments
- JS Variables
- JS var
- JS let
- JS const
- JS var Vs let Vs const
- JS Operators
- JS Arithmetic Operators
- JS Assignment Operators
- JS Comparison Operators
- JS Logical Operators
- JS Bitwise Operators
- JS Ternary Operator
- JS Operator Precedence
- JS Data Types
- JS typeof
- JS Conditional Statements
- JS Conditional Statement
- JS if Statement
- JS if...else Statement
- JS switch Statement
- JS Loops
- JS for Loop
- JS while Loop
- JS do...while Loop
- JS break Statement
- JS continue Statement
- JS break Vs. continue
- JavaScript Popup Boxes
- JS Dialog Box
- JS alert Box
- JS confirm Box
- JS prompt Box
- JavaScript Functions
- JS Functions
- JS setTimeout() Method
- JS setInterval() Method
- JavaScript Events
- JS Events
- JS onclick Event
- JS onload Event
- JS Mouse Events
- JS onreset Event
- JS onsubmit Event
- JavaScript Arrays
- JS Array
- JS Find Length of Array
- JS Add Elements at Beginning
- JS Add Element at End
- JS Remove First Element
- JS Remove Last Element
- JS Get First Index
- JS Get Last Index
- JS Reverse an Array
- JS Sort an Array
- JS Concatenate Arrays
- JS join()
- JS toString()
- JS from()
- JS Check if Value Exists
- JS Check if Array
- JS Slice an Array
- JS splice()
- JS find()
- JS findIndex()
- JS entries()
- JS every()
- JS fill()
- JS filter()
- JS forEach()
- JS map()
- JavaScript Strings
- JS String
- JS Length of String
- JS Convert to Lowercase
- JS Convert to Uppercase
- JS String Concatenation
- JS search()
- JS indexOf()
- JS search() Vs. indexOf()
- JS match()
- JS match() Vs. search()
- JS replace()
- JS toString()
- JS String()
- JS includes()
- JS substr()
- JS Slice String
- JS charAt()
- JS repeat()
- JS split()
- JS charCodeAt()
- JS fromCharCode()
- JS startsWith()
- JS endsWith()
- JS trim()
- JS lastIndexOf()
- JavaScript Objects
- JS Objects
- JS Boolean Object
- JavaScript Math/Number
- JS Math Object
- JS Math.abs()
- JS Math.max()
- JS Math.min()
- JS Math.pow()
- JS Math.sqrt()
- JS Math.cbrt()
- JS Math.round()
- JS Math.ceil()
- JS Math.floor()
- JS Math.trunc
- JS toFixed()
- JS toPrecision()
- JS Math.random()
- JS Math.sign()
- JS Number.isInteger()
- JS NaN
- JS Number()
- JS parseInt()
- JS parseFloat()
- JavaScript Date and Time
- JS Date and Time
- JS Date()
- JS getFullYear()
- JS getMonth()
- JS getDate()
- JS getDay()
- JS getHours()
- JS getMinutes()
- JS getSeconds()
- JS getMilliseconds()
- JS getTime()
- JS getUTCFullYear()
- JS getUTCMonth()
- JS getUTCDate()
- JS getUTCDay()
- JS getUTCHours()
- JS getUTCMinutes()
- JS getUTCSeconds()
- JS getUTCMilliseconds()
- JS toDateString()
- JS toLocaleDateString()
- JS toLocaleTimeString()
- JS toLocaleString()
- JS toUTCString()
- JS getTimezoneOffset()
- JS toISOString()
- JavaScript Browser Objects
- JS Browser Objects
- JS Window Object
- JS Navigator Object
- JS History Object
- JS Screen Object
- JS Location Object
- JavaScript Document Object
- JS Document Object Collection
- JS Document Object Properties
- JS Document Object Methods
- JS Document Object with Forms
- JavaScript DOM
- JS DOM
- JS DOM Nodes
- JS DOM Levels
- JS DOM Interfaces
- JavaScript Cookies
- JS Cookies
- JS Create/Delete Cookies
- JavaScript Regular Expression
- JS Regular Expression
- JS RegEx .
- JS RegEx \w and \W
- JS RegEx \d and \D
- JS RegEx \s and \S
- JS RegEx \b and \B
- JS RegEx \0
- JS RegEx \n
- JS RegEx \xxx
- JS RegEx \xdd
- JS RegEx Quantifiers
- JS RegEx test()
- JS RegEx lastIndex
- JS RegEx source
- JavaScript Advance
- JS Page Redirection
- JS Form Validation
- JS Validations
- JS Error Handling
- JS Exception Handling
- JS try-catch throw finally
- JS onerror Event
- JS Multimedia
- JS Animation
- JS Image Map
- JS Debugging
- JS Browser Detection
- JS Security
- JavaScript Misc
- JS innerHTML
- JS getElementById()
- JS getElementsByClassName()
- JS getElementsByName()
- JS getElementsByTagName()
- JS querySelector()
- JS querySelectorAll()
- JS document.write()
- JS console.log()
- JS instanceof
- JavaScript Programs
- JavaScript Programs
JavaScript Navigator Object
The navigator object in JavaScript is used to display information about the version and type of the browser.
The navigator object in JavaScript has the following three features:
- Collections
- Properties
- Methods
JavaScript Navigator Object Collections
The table given below describes the collections of the navigator object in JavaScript.
Collection | Description |
---|---|
plugins[] | returns a reference to all the embedded objects in the document |
mimeTypes | returns a collection of MIME types supported by client browser |
JavaScript Navigator Object Properties
The navigator object in JavaScript is used to retrieve properties of client browser. The following table describes properties of the navigator object in JavaScript.
Property | Description |
---|---|
appcodename | specifies the code name of the browser |
appname | specifies the name of the browser |
appversion | specifies the version of the browser being used |
cookieenabled | specifies whether the cookies are enabled or not in the browser |
platform | contains a string indicating the machine type for which the browser was compiled |
useragent | contains a string representing the value of the user-agent header sent by the client for the server in the http protocol |
JavaScript Navigator Object Properties Example
Here is an example demonstrates navigator object properties in JavaScript:
<!DOCTYPE HTML> <html> <head> <title>JavaScript Navigator Object Properties</title> <script type="text/javascript"> function dispNavigatorProperties() { with(document) { write("<b>appName = </b>") writeln(navigator.appName + "<br/><br/>") write("<b>appVersion = </b>") writeln(navigator.appVersion + "<br/><br/>") write("<b>appCodeName = </b>") writeln(navigator.appCodeName + "<br/><br/>") write("<b>platform = </b>") writeln(navigator.platform + " <br/><br/>") write("<b>userAgent = </b>") writeln(navigator.userAgent + "<br/><br/>") } } function dispExplorerProperties() { with(document) { write("<b>appName = </b>") writeln(navigator.appName + "<br/><br/>") write("<b>appVersion = </b>") writeln(navigator.appVersion + "<br/><br/>") write("<b>appCodeName = </b>") writeln(navigator.appCodeName + "<br/><br/>") write("<b>platform = </b>") writeln(navigator.platform + " <br/><br/>") write("<b>userAgent = </b>") writeln(navigator.userAgent + "<br/><br/>") write("<b>cookieEnabled = </b>") writeln(navigator.cookieEnabled + "<br/><br/>") } } function dispBrowserProperties() { if(navigator.appName == "Netscape") dispNavigatorProperties() else if(navigator.appName == "Microsoft Internet Explorer") dispExplorerProperties() } dispBrowserProperties() </script> </head> <body> </body> </html>
Here are some outputs of the above JavaScript navigator object properties example code. This is the snapshot of output produced in Google Chrome browser:
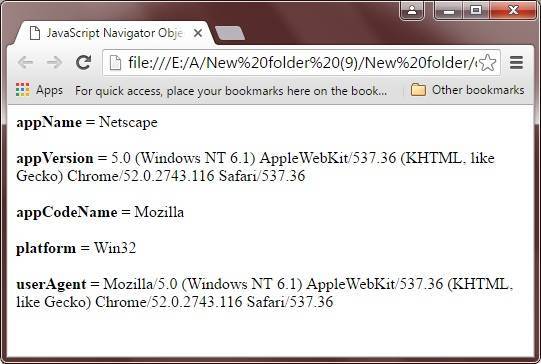
Here is the output produced in Mozilla Firefox browser:
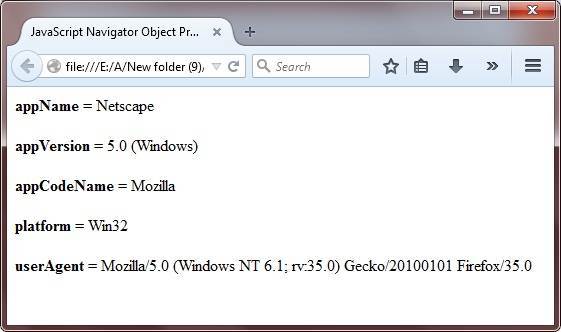
Here is the output produced in Safari browser:
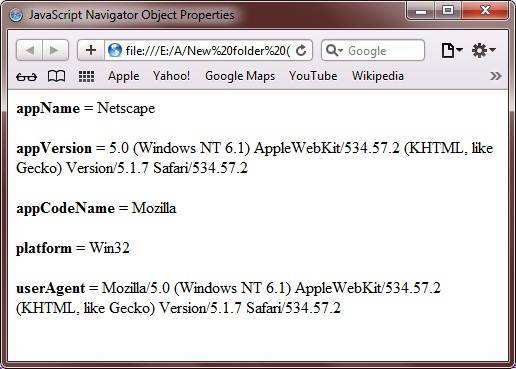
Here is the output produced in Microsoft Internet Explorer browser:
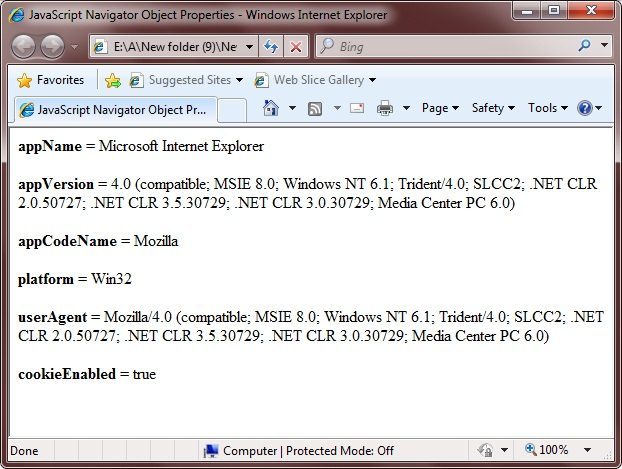
JavaScript Navigator Object Methods
The methods in the navigator object specify the action related to the browser. The table given below describes the methods of the navigator object in JavaScript.
Method | Description |
---|---|
javaEnabled() | tests whether Java is enabled or not |
taintEnabled() | determines whether data tainting is enabled or not |
Tainting prevents other scripts from passing information that should be secure and private, such as directory structures or suer session history
JavaScript Navigator Object Methods Example
Here is an example demonstrates navigator object methods in JavaScript:
<!DOCTYPE HTML> <html> <head> <title>JavaScript Navigator Object Methods</title> </head> <body> <h3>JavaScript Navigator Object Methods Example</h3> <script type="text/javascript"> document.write("<b>Java enabled: </b>" + navigator.javaEnabled() + "<br/>"); document.write("<b>Data tainting enabled: </b>" + navigator.taintEnabled()); </script> </body> </html>
Here are some sample outputs produced by above navigator object methods example code in JavaScript. This is the output produced in Google Chrome browser:
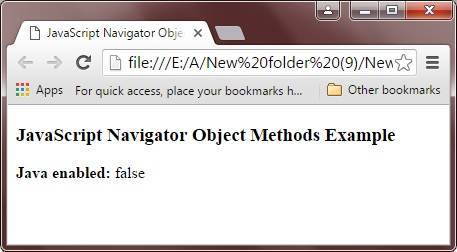
Here is the output produced in Mozilla Firefox browser:
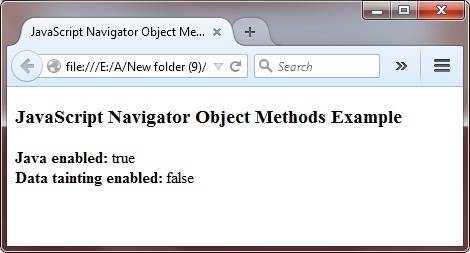
Here is the output produced in Safari browser:
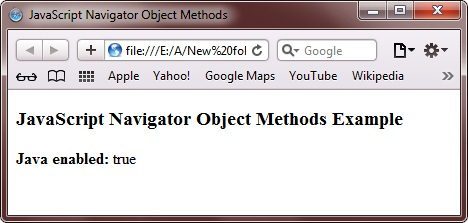
Here is the output produced in Microsoft Internet Explorer browser:
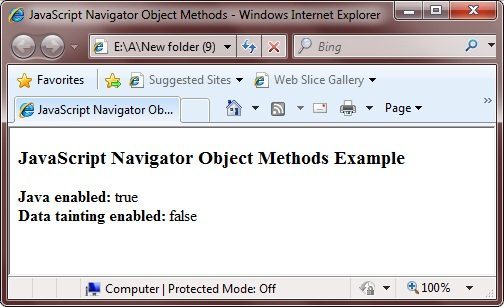
« Previous Tutorial Next Tutorial »